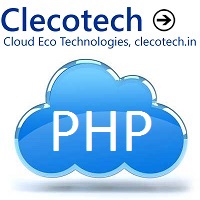
1. A method
2. An action
3. A submit button
<FORM METHOD=”post” ACTION=”yourscript.php”>
When you click a submission button in an HTML form, variables are sent to the script specified by the action via the specified method. The method can be either POST or GET. Variables passed from a form to a PHP script are placed in the superglobal called $_POST or $_GET, depending on the form method. In the next section, you’ll see how this works by creating an HTML form and accompanying PHP script that performs calculations, depending on the form input.
Creating a Calculation Form:
2. Type the following HTML
<head>
<title>My Own Calculator</title>
</head>
<body>
<form action = “calculate.php” method = “post”>
Value 1. <input type = text name = “calc1”><br/>
Value 2. <input type = text name = “calc2”><br/>
<input type = submit name = “calc” value = “Addition”>
<input type = submit name = “calc” value = “Subtract”>
<input type = submit name = “calc” value = “Division”>
<input type = submit name = “calc” value = “Multiply”>
</form>
</body>
</html>
3. Save the file as calculate_form.html and place it in the document root of your Web server.
Creating the Calculation Script
According to the form action in calculate_form.html, you need a script called calculate.php. The goal of this script is to accept the two values ($_POST[calc1] and $_POST[calc2]) and perform a calculation according to the value of $_POST[calc].
1. Open a new file in your text editor.
2. Start a PHP block and prepare an if statement that checks for the presence of the three values:
<?php
if(($_POST[‘calc1’] == “”) || ($_POST[‘calc2’] == “”) || ($_POST[‘calc’] == “”))
{
header(“Location:calculation.html”);
exit;
}
else if($_POST[‘calc’] == ‘Addition’)
{
$result = $_POST[‘calc1’] + $_POST[‘calc2’];
}
else if($_POST[‘calc’] == ‘Subtract’)
{
$result = $_POST[‘calc1’] – $_POST[‘calc2’];
}
else if($_POST[‘calc’] == ‘Division’)
{
$result = $_POST[‘calc1’] / $_POST[‘calc2’];
}
else if($_POST[‘calc’] == ‘Multiply’)
{
$result = $_POST[‘calc1’] * $_POST[‘calc2’];
}
?>
<html>
<head>
<title>My Own Calculator</title>
</head>
<body>
<p>THe result of the calculation is = <?=$result?></p>
</body>
</html>
3. Save the file with the name calculate.php, and place this file in the document root of your Web server.